In 2023, I discussed developing a clean architecture-inspired React application with MVVM at the code.talks conference.
A part of this talk was introducing common issues one could face during the development of an application and its lifecycle.
If you are interested, you can find the talk on YouTube. Just click on the following link:
YouTube
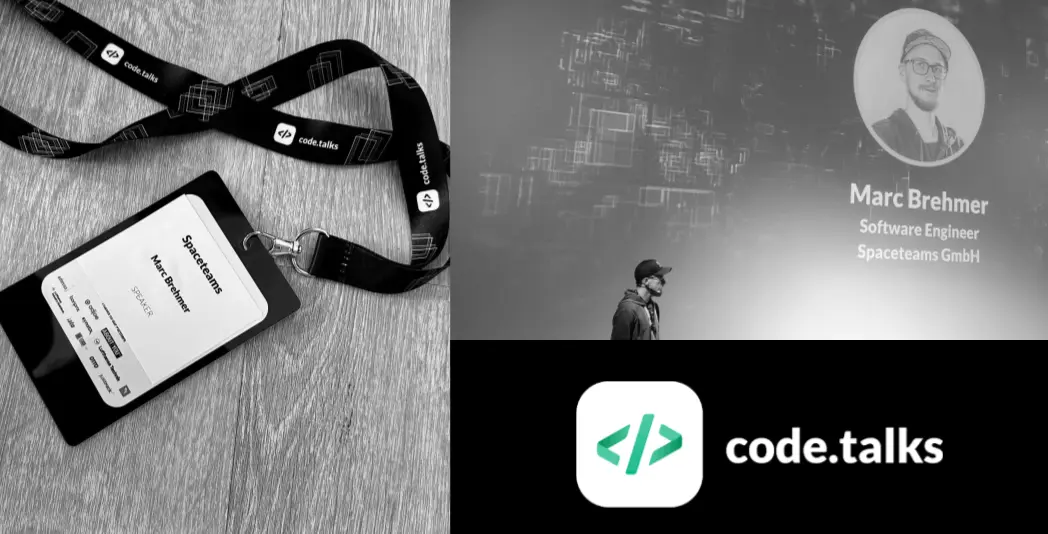
Issues of software development
Various issues can arise during the development of an application or later in its lifecycle, which includes maintaining it. If you are unaware of these issues and do not address them properly, you could face overspent budgets, delayed timelines, compromised quality, or, in the worst case, also a failed software project.
In the following sections, we examine a variety of issues, give examples of how they can occur, and discuss potential negative impacts. Finally, in the conclusion, we offer a hint on how to address these issues properly.
Code duplication
When we have a sequence of code that occurs more than once in our software, we are speaking of code duplications. These duplications can occur in various situations. The following list covers some examples:
- To save time, a developer could copy and paste code into multiple parts of the application instead of creating a reusable function.
- In a large and complex codebase, developers may write similar functions independently, which results in multiple versions of the same logic.
- Ineffective communication within a team can also lead to different developers writing the same code independently.
Duplicates have a negative impact on your software project. They can lead to:
- Increased maintenance costs since you need to track down and maintain duplicates whenever a change needs to be made, which is expensive and difficult.
- Higher risk of bugs, since a bug may be introduced in code duplicates. The bugs in the copies might go unnoticed at first and cause issues later.
- Reduced code quality since duplicated code is less organized and maintainable than well structured code.
- Reduced developer productivity because developers must spend considerable time maintaining and debugging code duplicates.
Tight coupling
Tight coupling occurs when different components of a software are highly dependent on one another.
Examples of tight couplings are:
- In a software system, we have a class
Class A
that directly depends on a different classClass B
. IfClass B
changes, we must modifyClass A
. - A specific concern is spread over multiple components instead of having its own component.
A tightly coupled system can have several downfalls:
- Adapting to evolving requirements or adding new features becomes challenging since changing a component can significantly impact others.
- Components can become less reusable if they're coupled with others.
- Tightly coupled systems are complex and can be hard to understand, which increases the risk of failure. If one component fails, it could cause others to fail as well, leading to a domino effect that could bring down the whole system.
- Testing becomes complex since components cannot be tested in isolation. Instead, one must also handle the coupled components while testing.
- Development costs can increase because changes to core components can also require updates to other components.
Scalability challenges
A scaling system is a software system that can adapt to increased load and traffic without sacrificing performance or reliability. These systems are developed to ensure they can handle demanding workloads, meet the needs of growing businesses, and provide reliable and efficient service to their users. These systems scale up to handle an increased workload and scale down when fewer resources are needed, reducing costs.
Examples of software systems where we could face scalability challenges are:
- An e-commerce website experiences a surge in traffic during a holiday sale. The server, which was not designed to scale, becomes overwhelmed, leading to slow response times or even downtimes.
- A social media platform stores all user data in a single database. As more users use the platform, the database becomes a bottleneck and causes performance issues. It may require a complex and costly migration to a more scalable solution.
Developing a scalable system can be challenging since it must scale easily and handle spikes without crashing or slowing down. Ensuring data consistency in such a system is an additional challenge because data distributed across multiple servers must remain consistent, which requires careful coordination between the servers.
The more complex and distributed a software system becomes, the more vulnerable it becomes to security attacks or breaches. Implementing security measures to protect the system and its users is essential, even though this might not be trivial.
It might be hard to tackle the named challenges, but addressing them is crucial to ensuring a software system's success.
Testing complexity
Testing complexity is the level of difficulty involved in testing a software system. Various factors, such as the size and complexity of the systems design and the availability of testing tools and resources, can influence this complexity.
Examples where we are faced with testing complexity are:
- Archiving a complete test coverage of a complex function with many conditional branches requires a large number of test cases. This makes testing time-consuming and prone to missing edge cases.
- Unit testing a single module in a tightly coupled system is difficult because it requires mocking all other modules on which our module depends. This is complex and time-consuming and leads to fragile tests that could break easily when code changes.
Complex systems require complex tests. Testing these systems takes longer, leading to increased costs and delayed releases. The code of a complex system is difficult to grasp thoroughly, which can lead to missing test cases and make complex systems more likely to contain bugs.
Addressing complexity during the software development process helps create less complex systems. Such systems are easier to test, and since they are easier to understand, it is more likely that no test cases are missed. Also, this makes the system less likely to contain bugs and can lead to cost savings, reduced risk, and improved quality.
Inconsistencies
Inconsistencies in a software system can occur when different parts of the system contradict each other.
Examples of inconsistencies are:
- Different naming conventions and coding styles are used for different parts of the codebase. This can make the code harder to read and understand, increase errors, and reduce code quality.
- A software consists of multiple modules that handle date and time differently. Some modules use local time, while others use UTC. This could lead to bugs when data is exchanged between these modules.
Several reasons can cause inconsistencies, like:
- Different conventions and coding styles.
- Different designs of implementations. An elementary example would be two methods for finding a text in a string. One method expects you to pass the string first, followed by the search term, while the other expects you to pass the search term first.
- Different UI interactions. For example, one dialog expects the user to hit enter, while another expects the user to click a button.
- Different UI designs. For example, various components of the UI could look completely different.
Inconsistencies can have several negative impacts on the software development, such as:
- Increasing costs are due to the difficulty of understanding, maintaining, and evolving the system.
- A higher risk of bugs, as identifying and fixing them can be challenging, especially in large and complex systems.
- Unexpected behaviors and errors decrease the quality of the software.
To ensure consistency, teams must properly communicate, find a consistent coding style, and agree on a uniform UI and UX.
Difficulty in migration
Migrating a software system to a new platform, environment, component, layer, or technology can be challenging. The difficulty level depends on various factors, such as the size and complexity of the system, the quality of its design, the availability of migration tools and resources, and the degree of coupling between its components.
Examples where we could face difficulty in migration are:
- You must migrate a legacy system with outdated technology to a modern platform. The system is tightly coupled, making isolating and migrating individual components difficult. This could lead to a lengthy and error-prone migration process.
- Migrating a system to a new database could require significant changes to the code, data transformation, and extensive testing to ensure data integrity and functionality.
The migration of complex systems:
- Is more expensive compared to simple systems.
- Requires a thorough planning, more effort, and more resources.
- Increase the risk of downtime, which can disrupt business operations and affect revenue.
- Can introduce new bugs into the system, reducing its quality and delaying the release of new features and updates.
Reduced maintainability
In this blog, we have covered various issues that could occur during software development, such as code duplications, tight couplings, and inconsistencies, among others. These issues can reduce a system's maintainability.
We could face reduced maintainability, for example, in these cases:
- A codebase with a high rate of code duplication. This would require us to make changes in multiple places, which increases the risk of introducing bugs and makes it harder to ensure that all instances of the duplicated code are updated consistently.
- A complex system with highly interdependent modules often requires changes to multiple other modules when making changes to one module. This increases the time and effort needed for maintenance and could also reduce the system's stability.
Reduced maintainability has several negative impacts on a software system:
- It can lead to increased costs, as a system may require more resources for maintenance.
- The system can be more likely to contain bugs, making identifying and fixing them even more challenging.
- Quality problems could exist, as improving and evolving a system can be difficult.
- Developing and releasing new features or updates could be delayed due to the time it takes to change the system.
Lack of flexibility
When we speak of a software system's lack of flexibility, we are speaking of the difficulty in adapting to new requirements. Different reasons can contribute to this problem, like tight couplings, a monolithic software design, or a lack of documentation.
To give a few examples where one could face a lack of flexibility:
- A tightly coupled system in which some of the business logic is hardcoded into the user interface. Changing the business rules is only possible by modifying the UI code, reducing the system's ability to adapt to new requirements.
- An application designed so that it cannot scale easily to handle increased load. Adding new features or improving the performance may require significant changes to the whole system. This makes the system less flexible and more complex to evolve.
A lack of flexibility has several negative impacts on software:
- It is expensive to change a system because changes may have unintended side effects and can be challenging to implement.
- New features and updates could take longer to release since changes need to be carefully planned and tested before they can be released.
- A system lacking flexibility is more likely to have quality issues because fixing bugs and improving the software is more difficult.
- Businesses that use inflexible software may face competitive disadvantages. They may be unable to respond to market changes quickly or meet their customers' needs.
Conclusion
Various issues could occur while developing software or during its lifecycle. If these issues are not properly addressed, they could lead to unsuccessful software projects characterized by overspent budgets, delayed timelines, and compromised quality.
Code duplications can increase maintenance costs and introduce bugs, while tight couplings negatively impact adaptability and innovation. These issues are not only technical challenges but can also translate into significant financial and operational setbacks. Scalability challenges, testing complexity, inconsistencies, migration difficulties, reduced maintainability, and a lack of flexibility further increase these problems.
Since we are aware of these issues, we can take measures to avoid them. A good way is to adopt an architectural pattern. An architectural pattern is a blueprint for designing software systems that are resilient against the issues discussed in this post. There are various architectural patterns available to choose from. A good example is the Clean Architecture pattern, which promotes principles such as separation of concerns, modularity, and encapsulation. It enables developers to create systems that are easier to maintain, scale, and evolve.
You can find a detailed explanation of Clean Architecture in our next blog post: Clean Architecture: A Deep Dive into Structured Software Design.
Overall, it is vital to be aware of the issues mentioned in this blog post. If you know them, you can adequately address them and avoid overly expensive or failing software projects.